Managing Multiple Basket Items as a Unit
Step 1: Adding a Composite Product to Basket
When adding the main product and options to the basket we’ll need some way to tell the “master order line” from the “sub order lines”.
We’ll use dynamic order properties to enrich the order lines for the required information linking each product option line to a master order line containing the main product; our MacBook Air 11 inch.
With that in mind here’s an example of adding the products to the basket.
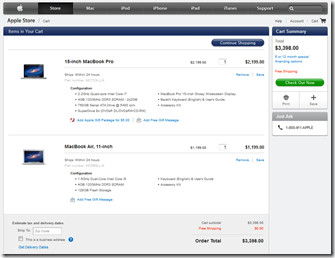
Ucommerce.Api.ITransactionLibrary transactionLibrary = ObjectFactory.Instance.Resolve<ITransactionLibrary>(); Ucommerce.EntitiesV2.PurchaseOrder basket = transactionLibrary.GetBasket(false); // Add and Mark the orderline as master Ucommerce.EntitiesV2.OrderLine mainOrderLine = transactionLibrary.AddToBasket(1, "Sku", null, addToExistingLine: false); mainOrderLine["master"] = true.ToString(); // Save basket to generate ids for the order line basket.Save(); // Add each product option to the basket posted by your model via MVC or WebAPI. Each key consists of a SKU unit that needs to be added foreach (var option in model.SelectedOptions) { // Add the option order line Ucommerce.EntitiesV2.OrderLine subOrderLine = transactionLibrary.AddToBasket(1, option.Sku, option.VariantSku, addToExistingLine: false); // Add a reference back to the master order line subOrderLine["masterOrderlineId"] = mainOrderLine.OrderLineId.ToString(); } // Execute the basket pipeline to recalculate basket transactionLibrary.ExecuteBasketPipeline();
Now we’ve got all the items added to the basket let’s move on to displaying the items in a nice way on the basket page.
Step 2: Displaying the Composite Product
Now we’ll need to leverage that extra information we added in the previous step to group the items together.
Ucommerce.Api.ITransactionLibrary transactionLibrary = ObjectFactory.Instance.Resolve<ITransactionLibrary>(); Ucommerce.EntitiesV2.PurchaseOrder basket = transactionLibrary.GetBasket(false); //Find all master orderlines IList<Ucommerce.EntitiesV2.OrderLine> orderLines = basket.OrderLines.Where(x => x.OrderProperties.Any(y => y.Key == "master" && y.Value == true.ToString())).ToList(); foreach (var mainOrderLine in orderLines) { //find sub orderlines var subOrderLines = basket.OrderLines.Where(x => x.OrderProperties.Any(y => y.Key == "masterOrderLineId" && y.Value == mainOrderLine.OrderLineId.ToString())).ToList(); }
Here’s what the basket looks like when Umbraco and Razor renders it:
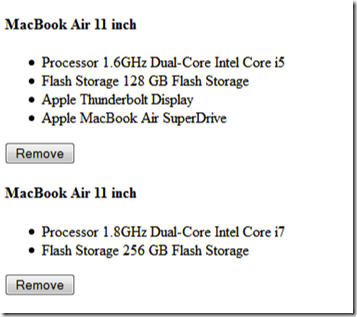
Step 3: Remove a Composite Product
Next we’ll want to let the customer remove composite items from the basket by remove the master order line along with any sub order lines it might have.
Ucommerce.Api.ITransactionLibrary transactionLibrary = ObjectFactory.Instance.Resolve<ITransactionLibrary>(); Ucommerce.EntitiesV2.PurchaseOrder basket = transactionLibrary.GetBasket(false); //when clicking to remove the id of the orderline id containing the main orderline is passed in. int masterOrderLineId = 1337; // Find all sub order lines for master var subOrderlines = basket.OrderLines.Where(x => x.OrderProperties.Any(y => y.Key == "masterOrderLineId" && y.Value == masterOrderLineId.ToString())).ToList(); // Remove sub order lines from basket foreach (var subOrderLine in subOrderlines) { basket.RemoveOrderLine(subOrderLine); } // Now remove the master order line OrderLine masterOrderLine = basket.OrderLines.Single(x => x.OrderLineId == masterOrderLineId); basket.RemoveOrderLine(masterOrderLine); // Recalculate basket transactionLibrary.ExecuteBasketPipeline();
Summary
Managing multiple items as one in the basket is handy when working with complex product options like we did for the MacBook Air just now. At all times should customers be certain as to what they’re buying and managing the items should be as straightforward as for “simple items”.
Combining multiple items into one visually is a matter of adding a bit of extra information to tie the items together and doing a clever presentation of the items.
All the internal calculations and stock management stay the same behind the scenes so you just have to worry about doing a nice presentation of the basket.