Building a Custom Shipping Method Service
Shipping and shipping costs in Ucommerce are calculated using the interface Ucommerce.Transactions.Shipping.IShippingMethodService
It allows for a single shipment with the orderlines and the order as the context to calculate what the costs should be.
Every shipping method available for a customer needs to be configured with such a service.
Out of the box, the default implementation will use a single price point configured on the individual shipping methods as the costs. However you might want a more dynamic calculation such as weight based shipping.
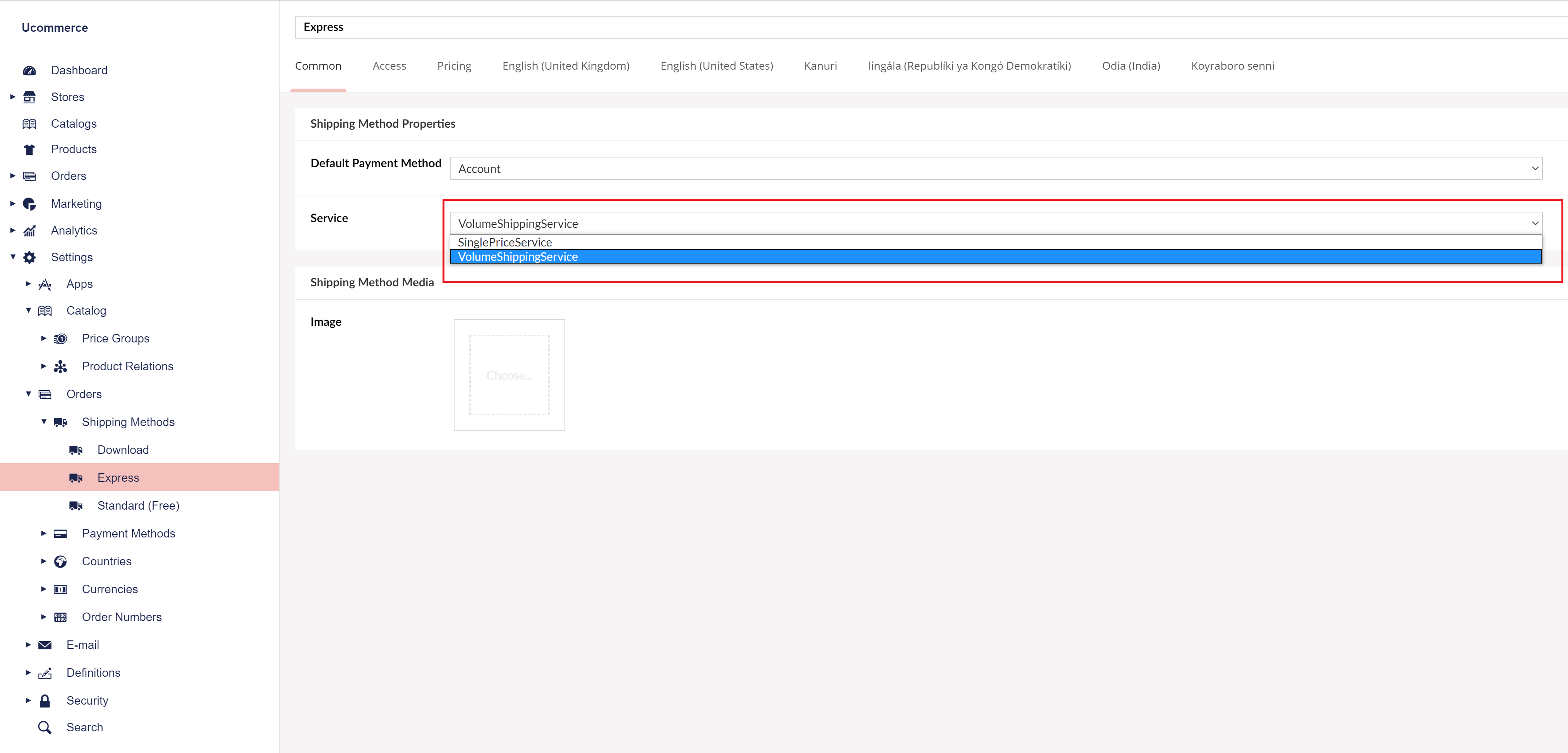
The Framework
Ucommerce will automatically take care of calling your implementation once it has been configured via our DI container. We will show how to do that in a little bit. Shipping costs are calculated in the basket pipeline every time the customer modifies the content of his order. This is great as it will always reflect the right price.
Ucommerce.Transactions.Shipping.IShippingMethodService
Description
Interface for calculating shipping fees
Methods
CalculateShippingPrice
- Description
Calculates the shipping fee for a particular Shipment -
Arguments
Ucommerce.EntitiesV2.Shipment
shipmentUcommerce.EntitiesV2.PriceGroup
priceGroup
- Return type
Ucommerce.Money
Volume Based Shipping Method Service
Volume and weight-based shipping pricing are probably the most common shipping scenarios for an online store so let's try and build one of them as an example.
As you can see from the specs above, you just need to implement a single method to get it going.
As discussed in Shipping Method Explained each shipping method can be configured to available only to certain stores and for shipment only to certain countries.
CalculateShippingPrice Method
public class Shipping : Ucommerce.Transactions.Shipping.IShippingMethodService { public void ShippingMethod() {} public Money CalculateShippingPrice(Ucommerce.EntitiesV2.Shipment shipment, Ucommerce.EntitiesV2.PriceGroup priceGroup) { // First sum up the total weight for the shipment. // We're assumning that a custom order line property // was set on the order line prior when the product was added to the order line. decimal totalWeight = 0; foreach (OrderLine orderline in shipment.OrderLines) totalWeight += orderline.Quantity * Convert.ToDecimal(orderline["Weight"]); decimal shippingPrice = 0; if (totalWeight > 10) shippingPrice = 100; else if (totalWeight > 20) shippingPrice = 200; else shippingPrice = 300; // To instantiate a new Money object we need the currency, // which is set on the purchase order. To get the currency // we move through Shipment -> OrderLines -> PurchasrOrder -> Currency return new Ucommerce.Money(shippingPrice, shipment.PurchaseOrder.BillingCurrency.ISOCode); }
Of course the business rule in this particular case is very simplistic, but you get the idea.
Registering Your ShippingMethodService with Ucommerce
You must also register the service by adding a new file to Ucommerce/Apps/MyApp
called Shipping.config with the following component:
<configuration> <components> <component id="VolumeShippingService" service="Ucommerce.Transactions.Shipping.IShippingMethodService, Ucommerce" type="MyUCommerceApp.VolumeShippingMethod, MyUCommerceApp"/> </components> </configuration>
With the app updated you will now be able to select your new shipping method service in the UI:
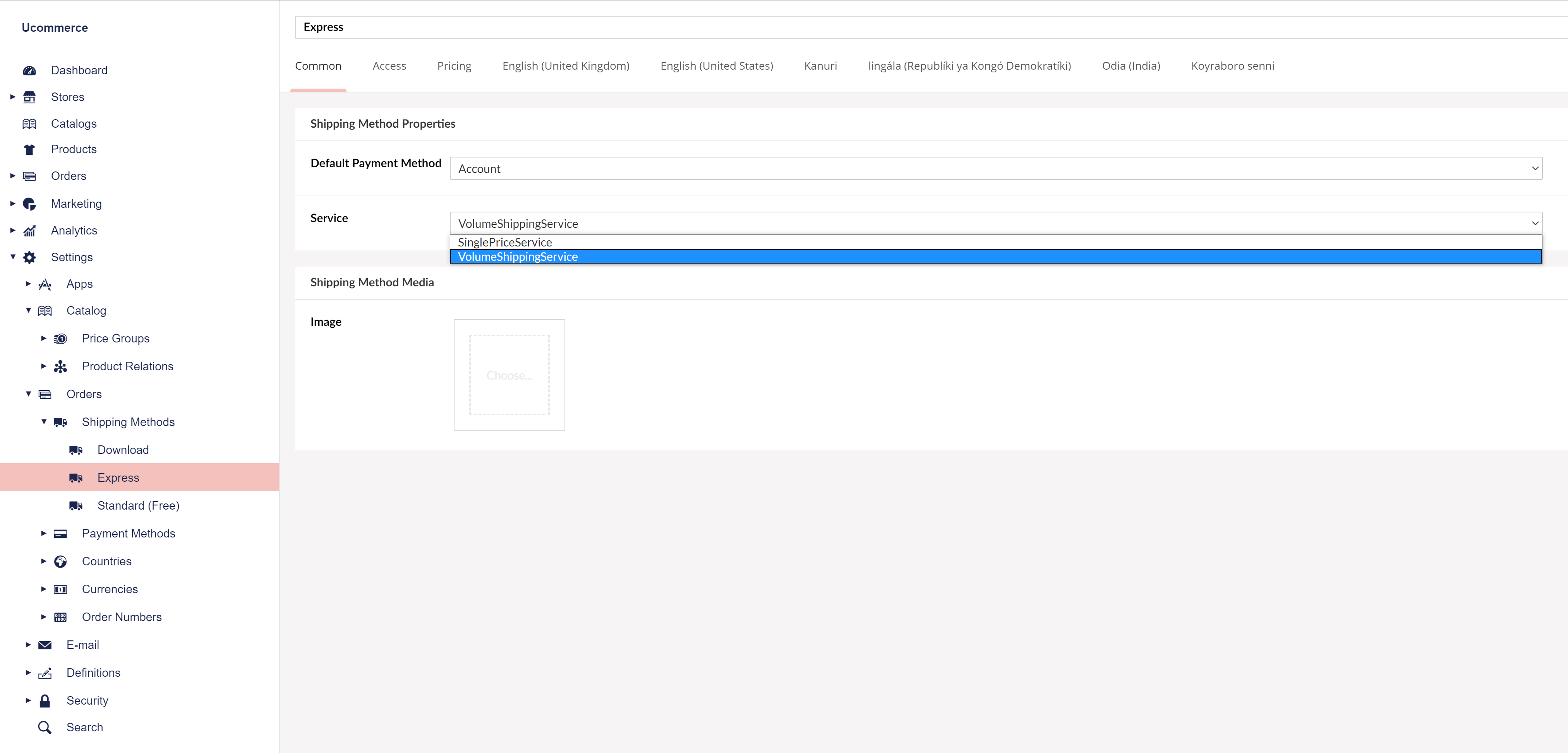
Summing It All Up
Creating your own shipping method service to handle custom calculations takes as little effort as implementing a single interface with a single method. Once it’s registered with Ucommerce users take over and set up the shipping method to their liking.
If you would like more information about Shipping Method please take a look at the article Shipping Methods Explained.