Integrating Ucommerce with a Payment Provider
You will learn how to create a Payment Method Service, which integrates Ucommerce with an online payment provider such as Sage, DIBS, PayPal, Cyber Source, or another provider used during checkout.
Implement Interface IPaymentMethodService
The IPaymentMethodService interface gives you full control over the behavior of the service. Alternatively you can inherit from AbstractPaymentService, which gives a default implementation for ProcessPaymentRequest, which will give you a properly initialized Payment.
Ucommerce.Transactions.Payments.IPaymentMethodService
Description
Interface for implementing a Payment Method Service for payment processing.
Methods
RequestPayment
- Description
Requests payment from backing service. -
Arguments
Ucommerce.Transactions.Payments.PaymentRequest
request
- Return type
Ucommerce.EntitiesV2.Payment
ProcessPaymentRequest
- Description
Processes the payment locally once the request is completed. -
Arguments
Ucommerce.Transactions.Payments.PaymentRequest
request
- Return type
Ucommerce.EntitiesV2.Payment
AcquirePayment
- Description
Acquires the payment. -
Arguments
Ucommerce.EntitiesV2.Payment
paymentToAcquire
- Return type
Ucommerce.EntitiesV2.Payment
CancelPayment
- Description
Cancels the payment. -
Arguments
Ucommerce.EntitiesV2.Payment
paymentToCancel
- Return type
Ucommerce.EntitiesV2.Payment
CalculatePaymentFee
- Description
Calculates the fee for a given PaymentMethod. -
Arguments
Ucommerce.Transactions.Payments.PaymentRequest
request
- Return type
Ucommerce.Money
Implement RequestPayment(PaymentRequest)
RequestPayment can be used in one of two ways: 1) Either to redirect the customer to the remote server handling payment information there, e.g. credit card information, or 2) to simply send payment information received on your server directly to the provider.
1)
public Payment RequestPayment(PaymentRequest request) { // Package up information for the backend service, // in the form of a http request // Redirect customer to backend service with // the needed information // Payment would be set pending authorization status (the status will have to be added to the Ucommerce_PaymentStatus table) return new Payment(); }
2)
public Payment RequestPayment(PaymentRequest request) { // Processing is happening synchronously so we just // call the backend service with ProcessPaymentRequest return ProcessPaymentRequest(request); }
Implement ProcessPaymentRequest(PaymentRequest)
ProcessPaymentRequest is used to handle any processing before or callbacks from the remote server in the case of a payment processing being handled exclusively by a remote party.
1) In the case of a callback scenario you use ProcessPaymentRequest to handle the callback and do any needed processing like updating order status. To receive the callback an endpoint needs to be exposed to the remote party such an http handler or a module. The handler or module will call ProcessPaymentRequest to complete the payment.
public Payment ProcessPaymentRequest(PaymentRequest request) { // Update payment according to received status from remote // party, e.g. Accepted, Declined // Grab any transaction ids and put them on the payment before // returning the Payment return new Payment(); }
2) For a synchronous scenario ProcessPaymentRequest would be used to perform the query to the backing system and create a new Payment according to the result received.
public Payment RequestPayment(PaymentRequest request) { // Package up information for the backend service, // in the form of a http request // Redirect customer to backend service with // the needed information // Payment would be set pending authorization status return new Payment(); }
Implement AcquirePayment(Payment)
For legal reason you usually can’t withdraw money from the customer until you actually ship their order. AcquirePayment is meant for this very scenario where you first authorize the payment and later withdraw from their account.
AcquirePayment(Payment) is used for capturing the payment from the customer.
public Payment ProcessPaymentRequest(PaymentRequest request) { // Send request to remote party // Update payment according to received status from remote // party, e.g. Accepted, Declined // Grab any transaction ids and put them on the payment before // returning the Payment return new Payment(); }
Implement CancelPayment(Payment)
If you wish to cancel a payment with your payment method service you need to implement CancelPayment, and have it reach out the remote payment processor. Typical scenarios for cancelling a payment includes a customer return, cancelled order and typically requires you to handle two scenarios: Payment authorized but not capture and payment authorized and captured.
public Payment CancelPayment(Payment payment) { // Update payment according to received status from remote // party, e.g. Cancelled // Grab any transaction ids and put them on the payment before // returning the Payment return new Payment(); }
Implement CalculatePaymentFee(PaymentRequest)
If applicable, used for calculating the fee associated with the payment method.
public virtual Money CalculatePaymentFee(PaymentRequest request) { var order = request.PurchaseOrder; var paymentMethod = request.PaymentMethod; var fee = paymentMethod.GetFeeForCurrency(order.BillingCurrency); if (!order.SubTotal.HasValue) return new Money(0, new CultureInfo(Globalization.CultureCode), order.BillingCurrency); return new Money(order.SubTotal.Value * (paymentMethod.FeePercent / 100) + fee.Fee, new CultureInfo(Globalization.CultureCode), order.BillingCurrency); }
Optionally Implement IPaymentFactory Interface on Your Provider
You can create a payment placeholder for the choices made by a user and then later perform the actual payment request to the backend service.
If you need this functionality your service should implement the interface IPaymentFactory, which will be used for creating the new Payment.
/// <summary> /// Creates a new payment fully initialized /// </summary> /// <param name="request"></param> /// <returns></returns> public Payment CreatePayment(PaymentRequest request) { PurchaseOrder order = request.PurchaseOrder; var payment = new Payment { TransactionId = Guid.NewGuid().ToString(), PurchaseOrder = order, PaymentMethodName = request.PaymentMethod.Name, Created = DateTime.Now, PaymentMethod = request.PaymentMethod, Fee = CalculatePaymentFee(request).Value, FeePercentage = request.PaymentMethod.FeePercent, PaymentStatus = PaymentStatus.Get(1), Amount = request.Amount.Value }; return payment; }
Copy Assembly to Your Site
Copy MyUCommerceApp.Library.dll to the /bin folder of your site.
Setup Payment Method Service
**Register Your Payment Method Service **
Register your new payment method service by adding a new file called Payments.config to Ucommerce/Apps/MyApp
to make it available in the backend:
<configuration> <components> <component id="MyPaymentMethodService" service="Ucommerce.Transactions.Payments.IPaymentMethodService, Ucommerce" type="MyUcommerceApp.Library.MyPaymentService, MyUcommerceApp" /> </components> </configuration>
Configure Payment Method to Use Your Service
Configure Payment Method to use your new service in Ucommerce Back office.
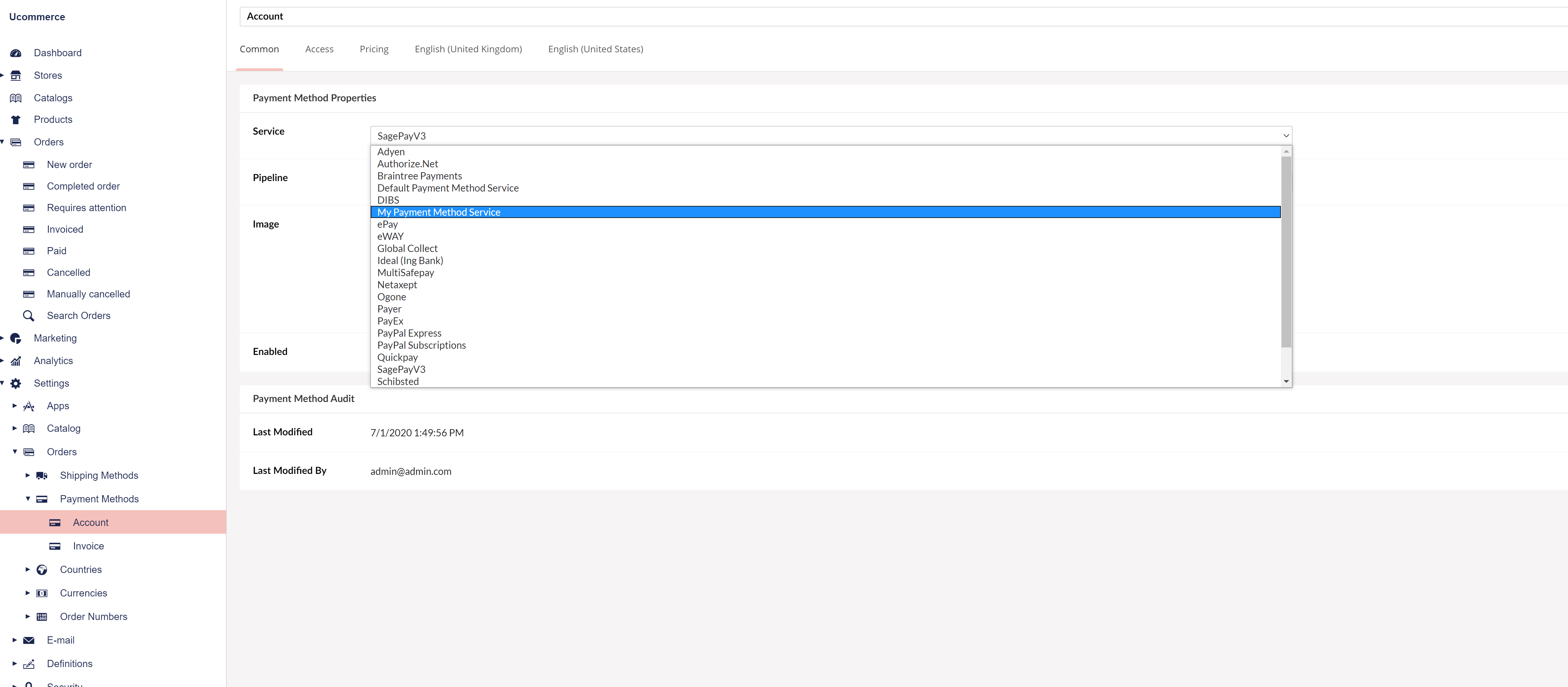
Request a payment for your integration
To execute your Payment Service directly from .NET code you use the following snippet:
Usage
public void ExecutePaymentMethodService(PaymentMethod paymentMethod, PurchaseOrder purchaseOrder, decimal amount) { var cultureInfo = new CultureInfo(Globalization.CultureCode); // Create a new payment request to var paymentRequest = new PaymentRequest( purchaseOrder, paymentMethod, new Money( amount, cultureInfo, purchaseOrder.BillingCurrency)); Payment payment = paymentMethod .GetPaymentMethodService() .RequestPayment(paymentRequest); }