Create a product using the API
If you want to create a product using the API, here’s how.
The class diagram for products looks like this:
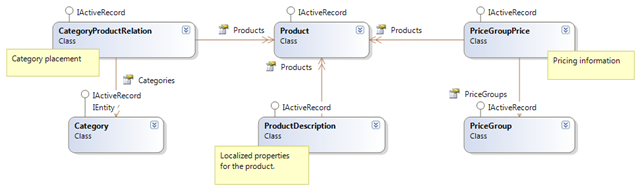
This might seem a bit verbose, but it’s all in favor of the flexible pricing and localization of products.
The data access is based on Active Record. If you haven’t already heart of that, check out the description on Wikipedia before reading any further.
Because localized descriptions, category placement and pricing information references the concrete product, we need to create the product itself first.
Before that, a short note on how to query the database for entites. If you want to find a specific entity,
you can use the static SingleOrDefault<T>(Func<T, bool>)
method. This will work for all entities:
var product = Product.SingleOrDefault(x => x.ProductId == 42);
If you want a collection of entities, use the Find
var products = Product.Find(x => x.ModifiedOn >= DateTime.Now.AddDays(-7));
You can also use more advanced Linq queries. Getting products modified within the last week can be retrieved like this:
var products = from p in Product.All() where p.ModifiedOn >= DateTime.Now.AddDays(-7) select p;
You can also do joins and so on.
The Product
The create the product, simply create a new instance of the UCommerce.Entites.Product class, set the properties, and finally call the save method.
var product = new Product { Sku = "SKU-123456", Name = "My Product", AllowOrdering = true, DisplayOnSite = true, ProductDefinitionId = 1, // Set to an existing defintion ThumbnailImageMediaId = 1 // ID from Umbraco (nullable int) }; product.Save();
After calling the save method, the ProductId property will return the ID of the new product.
Localized Product Description
To add a localized description for the product, create a new instance of the UCommerce.Entities.ProductDescription class.
var description = new ProductDescription { ProductId = product.ProductId, CultureCode = "en-US", // or en-GB, da-DK etc. DisplayName = "My display name", ShortDescription = "My short description", LongDescription = "My long description" }; description.Save();
Like with the product, the ProductDescriptionId will return the new ID of the description after calling the save method.
Pricing Information
The save a price for a product, you find the ID of the price group you want the price to belong to. After that, you can create a new Price and ProductPrice.
var priceGroup = PriceGroup.SingleOrDefault(x => x.Name == "DKK"); productPrice = new ProductPrice() { MinimumQuantity = 1, Guid = Guid.NewGuid(), Product = product, Price = new Price() { Amount = yourPrice, Guid = Guid.NewGuid(), PriceGroup = priceGroup } }; product.ProductPrices.Add(productPrice);
Note the MinimumQuantity property on ProductPrice! This is due to our Tier Pricing engine. If you are not utilizing this, and you want to set a default price, simply set the value to 1 as in the example above. If you are looking to create tier prices, simply create multiple ProductPrices and Prices with the same Product and PriceGroup only a different MinimumQuantity and Amount. You can read more about our Tier Pricing engine here.
Category Association
Last, but not least, you probably want to add the product to one or more categories. This is done using the CategoryProductRelation class.
var category = Category.SingleOrDefault(x => x.Name == "Software"); var relation = new CategoryProductRelation { ProductId = product.ProductId, CategoryId = category.CategoryId }; relation.Save();
That’s it, the new product is ready to be sold!
Custom Properties
If you have added custom properties to a product using product definitions, you can access the properties simply by using the indexer on the product. Note that properties are always stored as strings (nvarchar), so you need to do your own casting, depending on the type of the property.
var property = product["MyProperty"]; property.Value = "New value"; property.Save();