Search Library
Search library in Ucommerce allows developers to easily integrate out of the box faceted search and other search facilities. In Ucommerce we provide a ravenDB instance along with the core platform where all objects in the catalog section is indexed. This allows for fast and easy search functionality that we've made even easier with our Search library.
Search in General
In Ucommerce we've made an abstraction from RavenDB which is why you only have to focus on the four primary pieces in our Search Foundation:
- SearchLibrary
- IFacetedQueryable
- Facet
- Documents
The SearchLibrary
is the out of the box experiance made for easy and general use.
Most implementation needs to only go through this library to get up and running.
Our demo store Avenue-clothing.com uses only the SearchLibrary for faceted search.
Take it for a spin at Avenue-Clothing.com
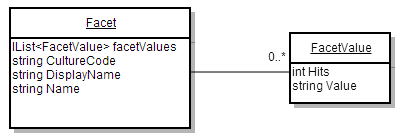
A Facet
in Ucommerce is a way of specifying both what you want and what you've already got.
This means that interacting with the SearchLibrary you both provide facets (of what you want)
and recieve facets back (which is a result set of possible facets based on what you've asked for).
In short and practical termonoligy: providing a list of facet is providing the list of choises the user just made.
Faceted Search
Faceted search in Ucommerce is provided out of the box from two methods in the search library.
IList<Facet> GetFacetsFor(Category category, IList<Facet> facets); IList<Product> GetProductsFor(Category category, IList<Facet> facets);
The two methods above are designed with browsing the catalog structure in mind. A natural flow in a webshop is showing products in a category, which is why both methods takes a category as an argument. The other bit of the methods is a List of facets corresponding to the choises the user have made.
In Avenue-Clothing.com The users choise are saved in a query string and transformed into facets which is then used to retrieve the remaining facets and products.
Getting Products from the SearchLibrary does not return Entities but documents, which in basic contains the same information but are fetched from the Raven database instead of a Sql Server. Those cannot be persisted into a SqlServer, so working with those should only be used for displaying products.
Getting Available Facets and Products
var category = SiteContext.Current.CatalogContext.CurrentCategory; var facets = SearchLibrary.GetFacetsFor(category, new List<Facet>()); var products = SearchLibrary.GetProductsFor(category, new List<Facet>());
Getting facets initial would usually look something similar to the above code. In this example I've used an empty list of facets corresponding to the initial load of the page.
Whenever a user clicks a facet, retrieving a subresult would look a little similar to this:
public IList<Facet> GetSelectedFacets() { var facetsForQuerying = new List<Facet>(); // The facets we return //Building a dictionary of facet keys and the selected values from querystring. var parameters = new Dictionary<string, string>(); foreach (var queryString in HttpContext.Current.Request.QueryString.AllKeys) { parameters[queryString] = HttpContext.Current.Request.QueryString[queryString]; } //Converting into facets foreach (var parameter in parameters) { var facet = new Facet(); facet.FacetValues = new List<FacetValue>(); facet.Name = parameter.Key; //In this example selected values are seperated by a '|' in the querystring value. foreach (var value in parameter.Value.Split(new[] { '|' }, StringSplitOptions.RemoveEmptyEntries)) { facet.FacetValues.Add(new FacetValue() { Value = value }); } facetsForQuerying.Add(facet); } return facetsForQuerying; }
The facets retrieved by the above code can be passed as a parameter instead of an empty list i used in the start.
Using FacetedQueryable
You also have the ability to do a completely custom query, not bound to a category. To achieve this you can work on a IFacetedQueryable retrieved from the searchLibrary.
//Normal way of using queryable. var query = SearchLibrary.FacetedQuery().Where(x => x.Variants.Any()); //adding facets to the query. IList<Facet> facetsForQuery = GetSelectedFacets(); query.WithFacets(facetsForQuery); //Getting facets for the query. IList<Facet> facets = query.ToFacets().ToList(); //Getting products for the query. IList<Product> products = query.ToList();