Building a Recently Browsed Items List with Ucommerce
A great tool for converting a customer is to display items they’ve previously looked at. One way to do it is by using persistent baskets, i.e. the basket contents remain the same even if a customer leaves and later returns to the store.
But what about the cases where the customer didn’t actually put anything in the basket, but we still want to be able to show some of the items she browsed recently?
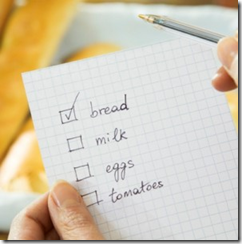
Approaches
The most obvious approach is to store SKUs in a cookie and present that list to the customer, but it makes for some pretty trivial use cases down the road, so let’s explore a different way of doing it: A custom basket.
Ucommerce comes with the ability to handle as many baskets per customer as you like so why not leverage this to create almost a wish list-like scenario. After all the recently browsed list is just a list of products that sure sounds like a basket?
Creating a New Basket
First up we need to create a new basket. By default Ucommerce will do one default basket where every item goes into, but we’ll need a second basket, which we can maintain ourselves.
Let’s do a little Razor, which will create that custom basket and tie it to the customer using a cookie:
@using UCommerce.Runtime; @using UCommerce.EntitiesV2; @using System.Web; @{ ProductCatalogGroup catalogGroup = SiteContext.Current.CatalogContext.CurrentCatalogSet; ProductCatalog catalog = ProductCatalog.All().Single(x => x.Name == SiteContext.Current.CatalogContext.CurrentCatalogName && x.ProductCatalogGroup == catalogGroup); Guid basketId = Request.Cookies["viewedProductsList"] != null ? new Guid(Request.Cookies["viewedProductsList"].Value) : Guid.Empty; PurchaseOrder viewedProductsList = basketId != Guid.Empty ? PurchaseOrder.All().SingleOrDefault(x => basketId != Guid.Empty && x.BasketId == basketId) : new PurchaseOrder(); // Initialize new order if (basketId == Guid.Empty) { viewedProductsList.OrderStatus = OrderStatus.Get((int)PurchaseOrder.StatusCode.Basket); viewedProductsList.ProductCatalogGroup = catalogGroup; viewedProductsList.BillingCurrency = catalog.PriceGroup.Currency; viewedProductsList.BasketId = Guid.NewGuid(); viewedProductsList.CreatedDate = DateTime.Now; HttpCookie cookie = new HttpCookie("viewedProductsList", viewedProductsList.BasketId.ToString()); cookie.Expires = DateTime.Now.AddDays(30); Response.Cookies.Add(cookie); } }
Adding a Product To The List
Once we have the custom basket set up we’ll just start adding items to it in the usual manner from the product page or whenever you deem that the customer showed enough interested to have a product on the recently browsed list.
// Add product being displayed Product product = Product.All().Single(x => x.Sku == "100-000-001" && x.VariantSku == "003"); viewedProductsList.AddProduct(catalog, product, 1); viewedProductsList.Save();
Displaying The Recently Browsed Items List
The third and final piece is to display the list. The code will basically have to look up the cookie tying the list to the customer, load the basket with contents, and display individual order lines to the customer. Simple stuff and here’s how to do it:
@using UCommerce.EntitiesV2; @using System.Web; @{ // Find the cookie containing the basketId Guid basketId = Request.Cookies["viewedProductsList"] == null ? Guid.Empty : new Guid(HttpContext.Current.Request.Cookies["viewedProductsList"].Value); // Load the list PurchaseOrder viewedProductsList = basketId != Guid.Empty ? PurchaseOrder.SingleOrDefault(x => x.BasketId == basketId) : new PurchaseOrder(); } @foreach (var orderLine in viewedProductsList.OrderLines.OrderBy(x => x.Quantity)) { <h3>@orderLine.ProductName</h3> <p>Bought @orderLine.Quantity times.</p> }
In Summary
We took a look at what it takes to maintain a separate custom basket for handling a recently browsed items type list. Using a custom basket instead of storing SKUs in a cookie has the added advantage of the extra information available when displaying the list to a customer.
Best of all it takes a trivial amount of code to get it up and running.
The code above sorts the list by quantity; the premise being that the product will have its quantity increased every time the customer looks at it thus we have a handy mechanism for determining the most popular items on the list. You can expand this to look at last modified dates on order lines to see when the customer was last interested in the product and so forth.
Finally the technique outline here is handy for scenarios like gift registries, wish lists, standard recurring orders, and other cases which require a custom list of products maintained either by the customer herself or by the store.