Creating a Custom Pipeline Task
You will learn how to create a custom pipeline task, which can be used to do custom task when Ucommerce executes the basket pipeline or any other pipeline for that matter.
In Ucommerce there's mainly 4 pipelines out of the box getting executed:
-
Basket pipeline
- this is executed each time a user updates the basket (puts stuff into it, adjusts the quantity or removes an item)
-
Checkout pipeline
- this is executed when the user has entered his credit card information and the order is being fullfilled
-
ToCompleted / ToCancelled pipeline
- When the order goes from new order to completed or cancelled.
In this example we'll create a pipeline task that will get executed in the Checkout pipeline. The purpose of this task is to tell a store administrator every time an order goes through the system. To achieve this we first need to:
- Create a visual studio project
- Create a class that implements
IPipelineTask<PurchaseOrder>
- Build and deploy the assemblies
- Configure the task in custom.config
On to the Code
Below is my class OrderCompletedTask
which implements IPipelineTask
namespace UCommerceCodeSamples.Pipelines { public class OrderCompletedTask : IPipelineTask<PurchaseOrder> { private readonly CommerceConfigurationProvider _provider; private readonly IEmailService _emailService; private readonly ICatalogContext _catalogContext; public OrderCompletedTask(CommerceConfigurationProvider provider, IEmailService emailService, ICatalogContext catalogContext) { _provider = provider; _emailService = emailService; _catalogContext = catalogContext; }
The next step to achieve this is to implement the method Execute
which Ucommerce will be responsible for
calling, whenever the basketpipeline is executed.
public PipelineExecutionResult Execute(PurchaseOrder subject) { var localization = new CustomGlobalization(_provider); localization.SetCulture(new CultureInfo(subject.CultureCode)); var queryStringParams = new Dictionary<string, string>(); queryStringParams.Add("orderguid", subject.OrderGuid.ToString()); queryStringParams.Add("orderid", subject.OrderId.ToString(CultureInfo.InvariantCulture)); var emailProfile = _catalogContext.CurrentCatalogGroup.EmailProfile; _emailService.Send(localization, emailProfile, "Orders", new MailAddress("[email protected]"), queryStringParams); return PipelineExecutionResult.Success; }
Configuring the Task
To make Ucommerce use the task we've just created we need to register it in Custom.Config which is the place for you to place your own components and overrides for Ucommerce. The file is found under Configuration/Custom.config in the Ucommerce folder under the cms application.
Below is the registration for the pipelineTask that i've put in the custom.config file.
This takes care of the configuration of the component itself. Now you need to add the task to a pipeline.
Configuring the Pipeline
When modifying an existing pipeline, you have three options, in the recommended order:
- Copy the entire pipeline to custom.config, and then make your modifications there.
- Make the modifications directly in the original pipeline config.
Copying the Pipeline Configuration to custom.config
In some cases, you need more complete control over the pipeline configuration.
And in that case, you can copy the entire pipeline component to custom.config.
It does, however, have the potential drawback, that any modification to the default pipeline made by new releases of Ucommerce, is not applied to the copy.
Please note, that any partial component configuration will also apply to components configured in custom.config.
Modifying the Pipeline File Directly
If you modify the pipeline directly, you lose the changes when upgrading or reinstalling the Ucommerce package. There it is recommended to use one of the two other options described above.
Below is the ToCompleted pipeline registered. Note that i've used the id registered for the task to register in the ToCompletedPipeLine.
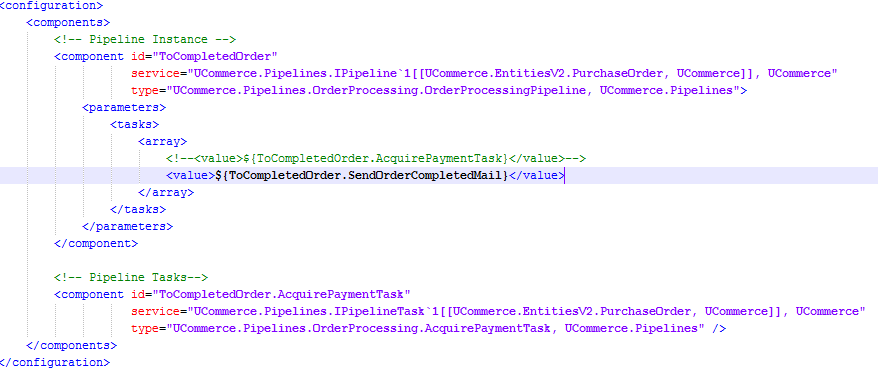
All there's left to do is build and deploy the assemblies into your Application's bin folder.
We've now extended the ToCompleted pipeline with a custom pipeline task.