Building Webshops with Umbraco, Razor, and the Ucommerce E-commerce Framework. Oh My!
Probably the most interesting new feature to come to Umbraco lately (for .NET developers anyway) is support for the Razor view engine. The Razor view engine was originally (sounds like it was year ago when in fact it only happened recently) introduced with MVC 3 to reduce friction when writing UI code.
XSLT, .NET, or Both
One of the first questions I’m asked when I demo Ucommerce to new partners is, “should we use XSLT or .NET user control to build our Ucommerce sites”.
My advice over the past couple of years has always been to use XSLT for static listings and roll out .NET for the more dynamic pieces like the check out flow or advanced product pickers.
It’s no secret that my background in .NET makes me whip out Visual Studio for almost everything, but XSLT does have some interesting attributes like the ability to build out a UI in no time and reskin existing UIs rapidly. That’s why we built the Ucommerce Demo Store in XSLT exclusively and that’s why I originally invested some time in getting to know it.
Now, however, my advice might be about to change. Read on and find out why.
Introducing Razor, Macros on Steroids!
The answer to make .NET devs as efficient creating UIs as XSLT gurus is Razor. While it won’t make developers better designers it will enable them to roll out UIs very rapidly and in a more fluent manner to boot.
The Razor view engine is basically an advanced parser, which enables you to write code much more fluently than what was possible with the WebForms view engine. Gone are the days of <%%> replaced by @.
Basically you can now write code like this in your macros and it will render properly inside your templates.
@using umbraco.cms.businesslogic.member @if (Member.GetCurrentMember() != null) { @string.Format("Hello there, {0}, nice to see you again!", Member.GetCurrentMember().LoginName) }
And this is what it looks like in Umbraco itself. Pretty straightforward, right? Create a new script file using CSHTML and then create a new macro, select the script you created previously, and you’re good to go.
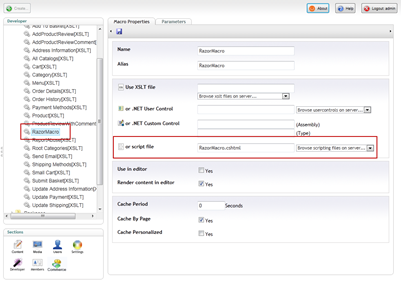
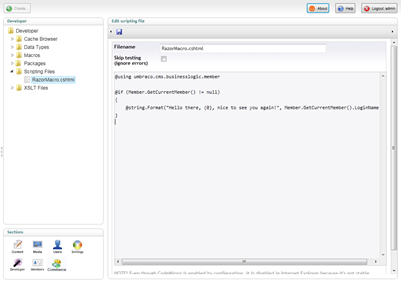
Ucommerce and Razor: Product Listing
So how does this work with Ucommerce? As it turns out, quite well. The Active Record pattern combined with LINQ to Ucommerce turns out to be a perfect fit for the Razor coding style. Lets see what it takes to get some products into the mix, shall we?
It’s very straightforward: Import the Ucommerce entities and query away!
@using UCommerce.EntitiesV2 @foreach (var product in Product.All()) { <h1>@product.Name</h1> }
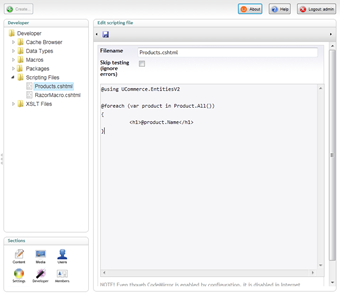
Not surprisingly, the code produces the following result.
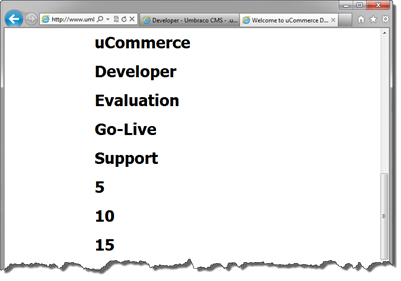
But what’s with the 5, 10, and 15? Turns out that the list contains both product, product families, and variants. Lets expand on the query a bit and only display the top level products and families.
Notice how the following query now has a Where clause on the call. What we’re doing is filtering the result to only grab the products we want. See how easy that is? Common operations like filtering, grouping, and even joining is supported which opens up for some very interesting scenarios.
@using UCommerce.EntitiesV2 @foreach (var product in Product.All().Where(x => x.ParentProductId == null)) { <h1>@product.Name</h1> }
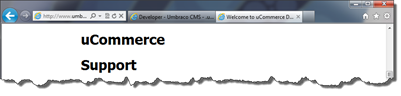
Simple Basket Page
How about another one just for kicks? What’s interesting here is that we’re leveraging the context aware API of Ucommerce to determine the basket given the current customer. Because the API deals with context you don’t have to worry about getting the right data, it’s just there ready to be consumed.
@using UCommerce.Runtime <table> <th>SKU</th><th>Name</th><th>Qty</th><th>Unit Price</th><th>Total</th> @foreach (var orderLine in SiteContext.Current.OrderContext.GetBasket().PurchaseOrder.OrderLines) { <tr> <td>@orderLine.Sku</td> <td>@orderLine.ProductName</td> <td>@orderLine.Quantity</td> <td>@orderLine.Price</td> <td>@orderLine.Total</td> </tr> } </table>
Again the code above is a full Razor macro in Umbraco, which is placed on a template to achieve the output below. With that we’ve got the beginnings of basket overview page. See how clean that is?
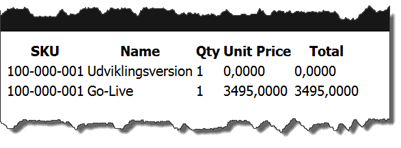
Summary
While Razor was introduced in Umbraco 4.6 it’s not really until 4.7 it’s become really usable. In testing Ucommerce with Umbraco 4.7 I found the combination of Razor and LINQ to Ucommerce to work surprisingly well. The flow inherent in Razor is carried over to the Ucommerce API. As a result the code flows naturally just the way we like it.
With Razor .NET developers start to see the same level of productivity which has been exclusive to the XSLT gurus when creating UIs. Not only that but the UI code is very readable and the full .NET framework is available without having to resort to XSLT extensions as is the case with XSLT today.